燃烧的火焰
操作系统 | 云服务/平台 | 技术难度 | 关注领域 |
---|---|---|---|
Android | Intermediate | Gaming Embedded |
任务目标
高通Adreno GPU具有强大的图形性能。
我希望做一个模拟的flame应用来演示这个性能,并使用Snapdragon探查器软件来分析CPU和GPU的性能使用情况。
应用程序启动时可以看到上面的图片,并且它会随着时间变换颜色
所需材料/所需清单/工具
源码/示例/可执行的应用程序
附加资料
构建/装配说明
以下为此项目中用到的工具
1. Android device with Snapdragon SDM660 processors installed the apk compiled by Android NDK, used to run apk and see flame display effect.
2.PC with Ubuntu 14.
3.PC with Windows 7.
4.Type-C data cable.
5.Adreno GPU SDK v5.0.
6.Android NDK r17b.
7.Apache Ant 1.9.14.
8.Snapdragon Profiler, a tool used to view apk performance indicators in real time.
部署项目
1. 根据此链接下载并安装到PC:https://developer.qualcomm.com/software/adreno-gpu-sdk
2. Download Android NDK r17b and install it to PC.
3. Download Apache Ant 1.9.14 and install it to PC.
4. Download Snapdragon Profiler from https://developer.qualcomm.com/software/snapdragon-profiler, and install it to PC.
5. 实现火焰的着色器
6. 在APK中调用火焰的着色器
7. 在APK中实现FPS的显示
8. 一步步实现需要的功能
9.选择安卓平台,连接设备并进行编译,生成APK
10.打开APK进行观赏
11.如果你关注性能指标,可以使用Snapdragon Profiler 来进行查看
12.如果没有问题,上传代码到github
工作流程
一、开始应该做些什么?
1、How to realize the flame shader?(如何实现火焰着色器)
In order to enable OpenGL to achieve flame drawing, we must first create a shader.
Vertex Shader:
#version 300 es
layout (location = 0) in vec4 vertex; // <vec2 position, vec2 texCoords>
out vec2 TexCoords;
out vec4 ParticleColor;
uniform mat4 projection;
uniform vec2 offset;
uniform vec4 color;
uniform float scale;
void main()
{
TexCoords = vertex.zw;
ParticleColor = color; //vec4(0.999995, 0.999995, 0.5, 0.0);
#ifdef ENABLE_VERTEX_OFFSET
gl_Position = projection * vec4(((vertex.xy - 0.5) * scale) + offset, 0.0, 1.0);
#else //!ENABLE_VERTEX_OFFSET
gl_Position = projection * vec4((vertex.xy * scale) + offset, 0.0, 1.0);
#endif //ENABLE_VERTEX_OFFSET
}
Fragment Shader:
#version 300 es
in vec2 TexCoords;
in vec4 ParticleColor;
out vec4 color;
uniform sampler2D sprite;
void main()
{
#if 1
color = (texture(sprite, TexCoords) * ParticleColor);
#else
color = ParticleColor;
#endif
}
This code creates the vertex shader and fragment shader respectively.
(这段代码分别创建了顶点着色器和片段着色器)
2、How to achieve the display of titles, menus, texts, etc?(如何实现标题、菜单、文本的显示)
Call the CFrmFontGLES and CFrmUserInterfaceGLES classes provided by the SDK framework to display the title, menu and text.(调用SDK框架提供的CFrmFontGLES、CFrmUserInterfaceGLES等类来实现标题、菜单和文字的显示)
// Create the font
m_pFont = new CFrmFontGLES();
if( FALSE == m_pFont->Create( "Samples/Fonts/Tuffy12.pak" ) )
{
FrmLogMessage("ERROR: create m_pFont failed\n");
return FALSE;
}
// Load the packed resources
CFrmPackedResourceGLES resource;
if( FALSE == resource.LoadFromFile( "Samples/Textures/Logo.pak" ) )
{
return FALSE;
}
// Create the logo texture
m_pLogoTexture = resource.GetTexture( "Logo" );
// Setup the user interface
if( FALSE == m_UserInterface.Initialize( m_pFont, g_strWindowTitle ) )
{
return FALSE;
}
m_UserInterface.AddOverlay( m_pLogoTexture->m_hTextureHandle, -5, -5, m_pLogoTexture->m_nWidth, m_pLogoTexture->m_nHeight );
m_UserInterface.AddTextString( (char *)"Press \200 for Help", 1.0f, -1.0f );
The above code creates the CFrmFontGLES and CFrmUserInterfaceGLES objects, respectively, where the CFrmUserInterfaceGLES object can call the AddOverlay and AddTextString methods to display icons and text.(上述代码分别建立了 CFrmFontGLES和 CFrmUserInterfaceGLES对象,其中CFrmUserInterfaceGLES 对象可以调用 AddOverlay和 AddTextString来实现图标和文字的显示)
3.How to display the frame rate?(如何实现帧率的显示)
Create a CFrmTimer class object and call the CFrmUserInterfaceGLES object in the render function to display the frame rate.(创建CFrmTimer类对象,并在Render中调用 CFrmUserInterfaceGLES对象来显示帧率)
// Update the timer
m_Timer.MarkFrame();
// Render the user interface
m_UserInterface.Render( m_Timer.GetFrameRate() );
4.How to compile and generate APK?(如何编译生成APK)
Compile thesource code(代码编译)
cd jni/
ndk-build -B
cd ..
Update the resource file(更新资源)
./InstallAssets.sh
android update project -p . -t android-20
Generate the APK file(使用ant命令打包生成APK)
ant debug
5.How to use Snapdragon Profiler software to analysis CPU and GPU utilization ?(如何使用 Snapdragon Profiler软件分析CPU和GPU的使用率)
1. Connect the phone to Snapdragon Profiler software.(将手机连接 Snapdragon Profiler软件)
2. Select the Realtime mode.(选择realtime模式)
3. Select CPU Utilization and GPU Utilization in process list, and the real-time utilization results will be displayed in the middle window.(选择process添加CPU Utilization和 GPU Utilization,实时的使用结果会显示在中间窗口)
6.How to use Snapdragon Profiler software to do OpenGL analysis?(如何使用 Snapdragon Profiler软件对OpenGL进行分析)
1.Connect the phone to Snapdragon Profiler software.(将手机连接 Snapdragon Profiler)
2.Change layout setting to OpenGL.(改变layout设置为OpenGL)
3.Select the Snapshot Capture mode.(选择Snapshot Capture模式)
4.Take Snapshot.(执行Take Snapshot操作)
使用说明
1、Download code from github according to the repository from “https://github.com/ThunderSoft-XA/Adreno-Burning-Flame.git”.(从此处下载源代码)
2、Install Adreno GPU SDK v5.0.(安装Adreno SDK)
3、Install Android NDK r17b.(安装Android NDK r17b)
4、Install Apache Ant 1.9.14.(安装Apache Ant1.9.14)
5、Install Snapdragon Profiler.(安装 Snapdragon Profiler)
6、Compile APK source code and install it to android device.(编译成APK并安装到安卓设备)
7、Open the APK, and you will enjoy it.(打开APK并欣赏它)
8、If you care about some performance indicators, you can use Snapdragon Profiler to view it.(如果关注性能指标,可以使用 Snapdragon Profiler查看)
贡献者信息
姓名 | 公司 |
---|---|
Zhen sunzhen@thundersoft.com |
Thundersoft |
Rong yangrong0925@thundersoft.com |
Thundersoft |
Wu kouzw0723@thundersoft.com |
Thundersoft |
Bo fangbo0326@thundersoft.com |
Thundersoft |
Qin yansq0930@thundersoft.com |
Thundersoft |
Xu |
Thundersoft |
>>浏览更多Qualcomm硬件案例:http://qualcomm.csdn.net/m/zone/qualcomm2016/project
Qualcomm 开发者专区是 Qualcomm 联合CSDN 共同打造的面向中国开发者的技术专区。致力于通过提供全球最新资讯和最多元的技术资源及支持,为开发者们打造全面一流的开发环境。本专区将以嵌入式、物联网、游戏开发、Qualcomm® 骁龙™处理器的软件优化等技术为核心,打造全面的开发者技术服务社区,为下一代高性能体验和设计带来更多的想法和灵感。
加入 Qualcomm 开发者专区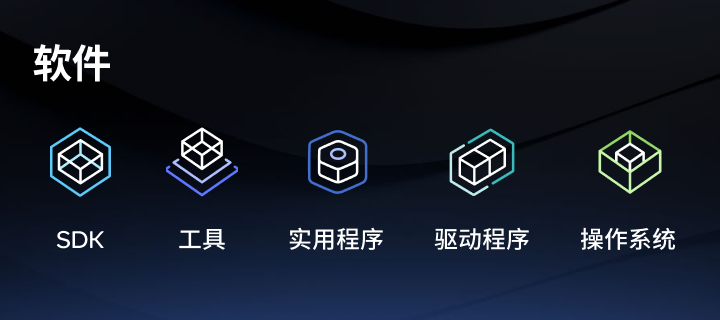
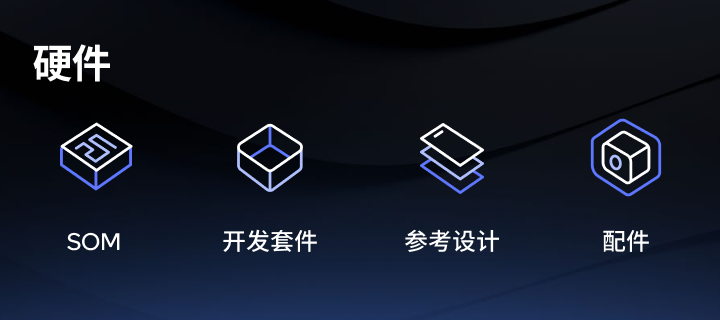
申请成为“Qualcomm荣誉技术大使”
“Qualcomm荣誉技术大使”是Qualcomm开发者社区对开发者用户技术能力与影响力的认证体现,该荣誉代表Qualcomm社区对用户贡献的认可与肯定。
立即申请招贤纳士
Qualcomm在中国的业务发展迅速,每年提供大量的技术岗位,分布在北京,上海,深圳等地。Qualcomm开发者社区是开发者藏龙卧虎之地,Qualcomm中国HR特别设立了招聘通道,欢迎开发者同学踊跃报名。
Qualcomm 活动 更多
5月27日
线上